The computer and machine language
A computer is a machine that accepts input data (instructions), processes it and then provides output data.
(output).
The instructions it reads are written in machine language, which is a binary language because it is composed of
only two characters: 0 and 1. Strings of 0 and 1 are called bits.
“The word "ciao" coded in binary:
01100011 01101001 01100001 01101111.“
In order to interact with computers, therefore, you should write instructions consisting of 0 and 1. To facilitate this process, you can interact with a computer using high level programming languages. High level means that the language is close to that of man. Java and C are examples of high-level language, In fact, many keywords in these languages correspond to words in English. But how can the computer understand these high-level instructions if it can only read 0 and 1? This is where the compilers help us.
“The compiler of a certain X language (X can be Java), is a program that reads the source program,
containing instructions written in that language, and, if there are no errors, turns it into an object program, that is to say
a program containing instructions written in machine language“
At that point the computer is able to read and run the object program.
What it means to program
Programming therefore consists in writing instructions for a particular language
programming, in files called sources. The set of source files is called source program..
We can write instructions using any text editor like a Notepad.
Once the source program is written, it is compiled, i.e. "transformed" into an object program,
written in machine language and ready to run.
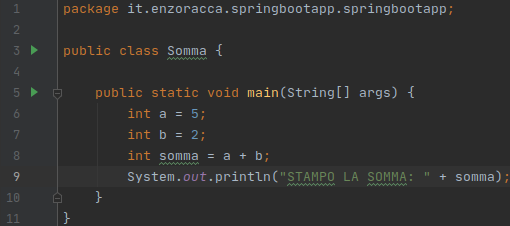
A program allows you to concretize the resolution of one or more algorithms.
“An algorithm is a finite sequence of steps needed to solve a problem. The same problem can also be solved with different
algorithms.“
Example of problem: Give in input 2 integers a and b, calculate the product of a and b without using the multiplication operator.
Algorithm:
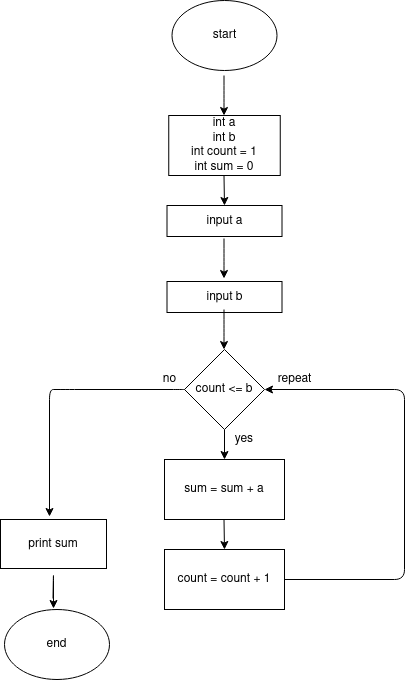
The flow chart or flow diagram (or also called block diagram), is not the only way to represent an algorithm; another way for example is to use a language
pseudo-code, i.e. halfway between human words and those of the programming language:
- int a, b, count = 1, sum = 0
- input a
- input b
- while count <= b do
4.1 sum = sum + a
4.2 count = count + 1 - end while
- output sum
The algorithm will then have to be written in the chosen programming language.
In the next lesson we will see how to write this algorithm in Java.
Types of programming paradigms
There are various types of programming paradigms, i.e. various ways of programming.
Each programming language uses one or more paradigms. For simplicity we will clarify the concepts of
procedural paradigm and the object paradigm.
In the procedural paradigm, a program can be divided into small subprograms called functions or procedures. The example of the previous algorithm can be enclosed in a product function, which can then be called in any point of the program.
In the image above we can see that 2 product and division functions have been created, call them later on at one point in the program. An example of procedural language is C.
The object-oriented programming is an evolution of the previous paradigm, it
brings to closer to representing the real world in an abstract way.
It consists of objects exchanging messages among themselves.
A class is an abstraction of a real world entity. An example of a class can be Person, Car, Company.
Each class has attributions. For example the class Person can have as attributes name, surname,
date of birth.
Also, in addition to attributes, each class has methods which are a bit like the functions of the paradigm
procedural. For example, the Person class could have the CalculateEta or SetName method.
An object is an instance of a class. I can have an object called Enzo of type Persona, an object
called Marco of type Person. Objects communicate with each other through methods.
In practice, just as we can define a variable named "a" of type whole, I can define
a variable named "Enzo" of type Person.
Since Java is an OOP language, we will go into the details of this paradigm in the following lessons.